- What Are Software Design Patterns?
- The Purpose of Software Design Patterns
- Applying Software Design Patterns Across Different Programming Languages
- Types of Software Design Patterns
- Commonly Used Software Design Patterns
- Advantages of Software Design Patterns
- Potential Drawbacks and Limitations of Using Design Patterns
- Conclusion
Ever wondered how some software projects run smoothly while others become a tangled mess? The secret often lies in something called software design patterns.
Let’s dive into the basics and purpose of these powerful tools.
What Are Software Design Patterns?
Imagine you’re building a house. You wouldn’t start from scratch each time, right? You’d use blueprints and templates to ensure everything fits together perfectly.
Similarly, in software development, design patterns are like these blueprints. They are proven solutions to common problems that developers face while creating software.
The Purpose of Software Design Patterns
- Consistency: They provide a consistent method for solving issues, making code easier to understand and maintain.
- Efficiency: By reusing these tried-and-tested solutions, developers save time and avoid pitfalls.
- Scalability: Good design patterns help in building software that can grow and adapt with ease.
Applying Software Design Patterns Across Different Programming Languages
Design patterns are like universal tools in a developer’s toolkit. They offer solutions that can be adapted and applied in various programming languages, making them versatile and broadly applicable. Here’s how design patterns transcend language boundaries:
1. Universal Concepts
Design patterns are based on abstract concepts rather than specific language features. For instance, the Singleton pattern ensures only one instance of a class exists, regardless of whether you’re coding in Java, Python, or C#. The core idea remains the same: control the instance creation.
2. Language Adaptability
Each programming language has its own syntax and features, but the fundamental principles of design patterns can be implemented in all. For example:
- Java: Uses classes and interfaces to implement patterns like Factory and Observer.
- Python: Leverages its dynamic nature to create patterns like Singleton and Strategy with minimal code.
- C++: Utilizes pointers and memory management to implement patterns like Prototype and Adapter.
3. Object-Oriented Principles
Most design patterns are built on object-oriented principles like inheritance, encapsulation, and polymorphism. These principles are present in almost all modern programming languages, allowing patterns to be applied widely.
For example, the Adapter pattern, which helps incompatible interfaces work together, can be applied using class inheritance or interface implementation in various languages.
4. Code Reusability
Patterns encourage code reusability. Once you understand a pattern, you can apply it in any language that supports the necessary programming constructs. This adaptability saves time and effort, as you can transfer your knowledge across different projects and languages.
5. Community and Documentation
Many programming languages have communities and documentation that provide guidelines and examples of how to implement design patterns.
For example, Java’s extensive libraries and frameworks often come with built-in support for patterns like MVC (Model-View-Controller). Similarly, Python’s documentation includes examples of using patterns in practical applications.
6. Cross-Platform Development
Design patterns are particularly useful in cross-platform development. They provide a consistent approach to solving problems, making it easier to maintain and manage codebases that span multiple platforms. For example, the Command pattern can be used to handle user inputs in both desktop and mobile applications.
Types of Software Design Patterns
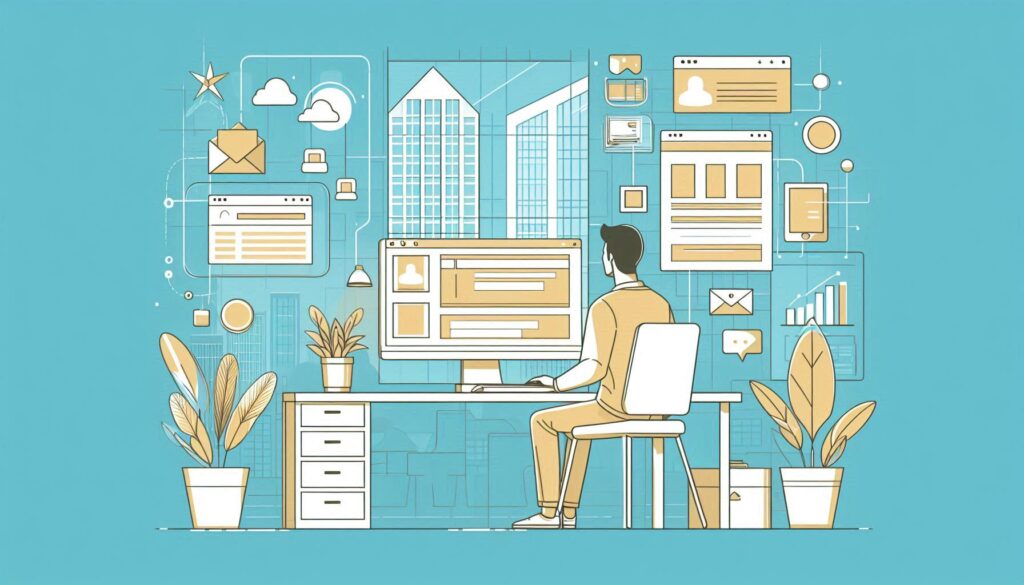
Software design patterns come in handy when building efficient, maintainable, and scalable software. Let’s explore the three main types: Creational, Structural, and Behavioral patterns.
Creational Patterns
Creational patterns deal with object creation mechanisms. They aim to simplify object instantiation and ensure that created objects are suitable for the task at hand.
- Singleton: Ensures a class has only one instance and provides a global point of access to it.
- Factory Method: Defines an interface for creating objects but lets subclasses alter the type of objects that will be created.
- Abstract Factory: Provides an interface for creating families of related or dependent objects without specifying their concrete classes.
- Builder: Separates the construction of a complex object from its representation, allowing the same construction process to create different representations.
- Prototype: Creates new objects by copying an existing object, known as the prototype.
Structural Patterns
Structural patterns deal with the composition of objects and classes. They help ensure that if one part changes, the entire structure does not break.
- Adapter: Allows incompatible interfaces to work together by converting the interface of a class into another interface expected by clients.
- Composite: Composes objects into tree structures to represent part-whole hierarchies. It lets clients treat individual objects and compositions of objects uniformly.
- Proxy: Provides a placeholder for another object to control access to it.
- Decorator: Attaches additional responsibilities to an object dynamically, offering a flexible alternative to subclassing for extending functionality.
- Facade: Provides a simplified interface to a complex subsystem, making the subsystem easier to use.
- Flyweight: Uses sharing to support a large number of fine-grained objects efficiently by minimizing memory usage.
Behavioral Patterns
Behavioral patterns focus on communication between objects. They help in assigning responsibilities between objects and managing algorithms and workflows.
- Observer: Defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically.
- Strategy: Defines a family of algorithms, encapsulates each one, and makes them interchangeable, allowing the algorithm to vary independently from clients that use it.
- Command: Encapsulates a request as an object, thereby allowing for parameterization of clients with queues, requests, and operations.
- Chain of Responsibility: Passes a request along a chain of handlers, where each handler either processes the request or passes it to the next handler.
- State: Allows an object to alter its behavior when its internal state changes, appearing to change the class of the object.
- Template Method: Defines the skeleton of an algorithm in a method, deferring some steps to subclasses.
- Mediator: Defines an object that encapsulates how a set of objects interact, promoting loose coupling by keeping objects from referring to each other explicitly.
- Visitor: Represents an operation to be performed on the elements of an object structure, allowing for new operations without changing the classes of the elements on which it operates.
Each of these patterns provides a tested solution to common software design issues, helping you build software that is more robust and easier to maintain.
Commonly Used Software Design Patterns
Some of the most frequently implemented design patterns in software development:
1. Singleton Pattern
This pattern ensures a class has only one instance and provides a global point of access to it. It’s commonly used in database connections and logging.
2. Factory Pattern
The Factory Method pattern defines an interface for creating an object, but lets subclasses decide which class to instantiate. It promotes loose coupling by eliminating the need to bind application-specific classes into the code.
3. Observer Pattern
This pattern defines a one-to-many dependency between objects. When one object (the subject) changes state, all its dependents (observers) are notified and updated automatically. It’s widely used in event handling systems like GUI toolkits.
4. Strategy Pattern
The Strategy pattern defines a family of algorithms, encapsulates each one, and makes them interchangeable. This allows the algorithm to vary independently from clients that use it. It’s often used in sorting and searching algorithms.
5. Decorator Pattern
This pattern attaches additional responsibilities to an object dynamically. It provides a flexible alternative to subclassing for extending functionality. You might see this in GUI frameworks where you want to add new features to components.
6. Adapter Pattern
The Adapter pattern allows otherwise incompatible classes to work together by converting the interface of a class into another interface clients expect. It’s useful when integrating new components into an old system.
7. Composite Pattern
The Composite pattern composes objects into tree structures to represent part-whole hierarchies. It lets clients treat individual objects and compositions of objects uniformly. This is common in systems that manage hierarchies of objects.
8. Command Pattern
This pattern encapsulates a request as an object, thereby allowing for parameterization of clients with queues, requests, and operations. It’s often used in GUI buttons and menu actions.
9. Proxy Pattern
The Proxy pattern provides a surrogate or placeholder for another object to control access to it. This can be useful for lazy initialization, access control, or logging.
10. MVC (Model-View-Controller) Pattern
MVC divides an application into three interconnected components: Model (data), View (UI), and Controller (business logic). This separation helps manage complexity and makes the application more modular.
These patterns are popular because they help solve common problems efficiently and effectively, making the software more reliable and easier to maintain.
Advantages of Software Design Patterns
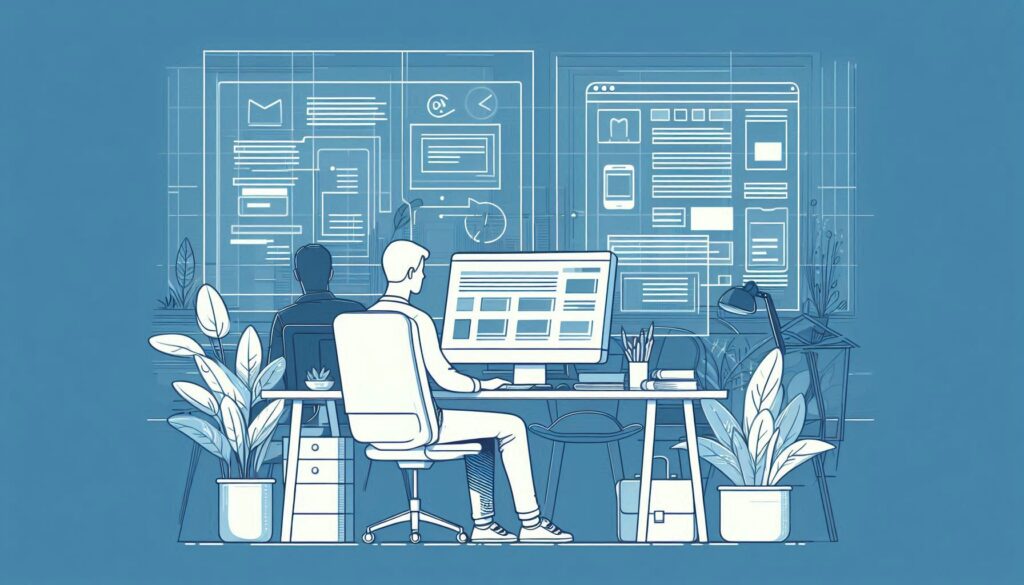
Software design patterns are like having a superhero team in your coding toolkit. They provide numerous benefits that can make your development process smoother and your code more robust. Let’s dive into the key advantages:
1. Improved Code Maintainability
Design patterns lead to cleaner, well-structured code. This makes it easier to understand, modify, and debug. Imagine trying to fix a bug in a codebase that’s a tangled mess versus one that’s neatly organized. The latter is much easier, right? Patterns ensure that your code remains maintainable over time.
2. Enhanced Scalability
As your project grows, design patterns help you scale without breaking a sweat. They provide a robust foundation that can handle increasing complexity. For instance, using a Composite pattern allows you to manage and manipulate hierarchical structures efficiently, making your codebase ready to grow.
3. Increased Reusability
Patterns encourage code reuse. Instead of writing new code for every problem, you can apply a pattern that has already solved similar issues. This not only saves time but also reduces the likelihood of errors. Think of it as using a reliable recipe to cook a familiar dish, ensuring consistency and quality.
4. Consistency in Codebase
Using design patterns brings consistency across your codebase, especially in team settings. Everyone speaks the same language, follows the same solutions, and understands the same structures. This makes collaboration smoother and onboarding new team members quicker.
5. Faster Development Time
By leveraging established patterns, you can develop software more quickly. You don’t have to spend time inventing solutions from scratch. Instead, you apply patterns that have been tested and proven effective. This can significantly accelerate your development cycles.
6. Easier Communication
Patterns provide a common vocabulary for developers. When you mention a “Singleton” or “Observer,” everyone knows what you’re talking about. This simplifies communication and ensures that everyone is on the same page, reducing misunderstandings and improving collaboration.
Potential Drawbacks and Limitations of Using Design Patterns
While software design patterns offer many benefits, they are not without potential drawbacks and limitations. Here are a few to keep in mind:
1. Overuse and Complexity
Design patterns can introduce unnecessary complexity if overused. Not every problem requires a pattern solution. Sometimes, simpler code is better. Over-engineering can make the code harder to read and maintain.
2. Misapplication
Using the wrong pattern for a specific problem can lead to inefficient and convoluted code. Misapplication often happens when developers try to force a pattern into a situation where it doesn’t fit well, leading to “square peg in a round hole” scenarios.
3. Learning Curve
For beginners, understanding and applying design patterns can be daunting. It takes time and experience to know when and how to use them effectively. Inexperienced developers might misuse patterns or apply them without fully grasping their intent.
4. Performance Overhead
Some design patterns can introduce performance overhead. For example, the Decorator pattern might add layers of indirection, and the Observer pattern might lead to excessive notifications if not managed properly.
5. Rigidity
Once a pattern is implemented, it can sometimes make the code more rigid and harder to change. This is particularly true if the pattern was chosen poorly or if the requirements evolve in unforeseen ways.
6. False Sense of Security
Relying too much on design patterns might give a false sense of security, making developers think they are immune to bad design choices. Patterns are helpful tools, but they do not replace good judgement and understanding of the problem at hand.
Conclusion
Software design patterns are like a well-oiled machine that makes your development process efficient, your code clean, and your projects scalable. They’re your go-to solutions for common problems, saving you time and reducing errors. So, next time you face a coding challenge, remember: there’s probably a pattern for that! Happy coding! 🎉
Hope this makes sense! Let me know if you want to dive deeper into any specific pattern.
READ MORE:
Top 7 GDPR Cookie Consent Plugins for WordPress
Open Source vs Proprietary: Understanding Key Differences